共计 2469 个字符,预计需要花费 7 分钟才能阅读完成。
redux 理解
学习文档
- 英文文档: https://redux.js.org/
- 中文文档: http://www.redux.org.cn/
- Github: https://github.com/reactjs/redux
redux 是什么
- redux 是一个专门用于做状态管理的JS库(不是 react 插件库)。
- 它可以用在 react, angular, vue 等项目中, 但基本与 react 配合使用。
- 作用: 集中式管理 react 应用中多个组件共享的状态。
什么情况下需要使用 redux
- 某个组件的状态,需要让其他组件可以随时拿到(共享)。
- 一个组件需要改变另一个组件的状态(通信)。
- 总体原则:能不用就不用, 如果不用比较吃力才考虑使用。
redux 工作流程
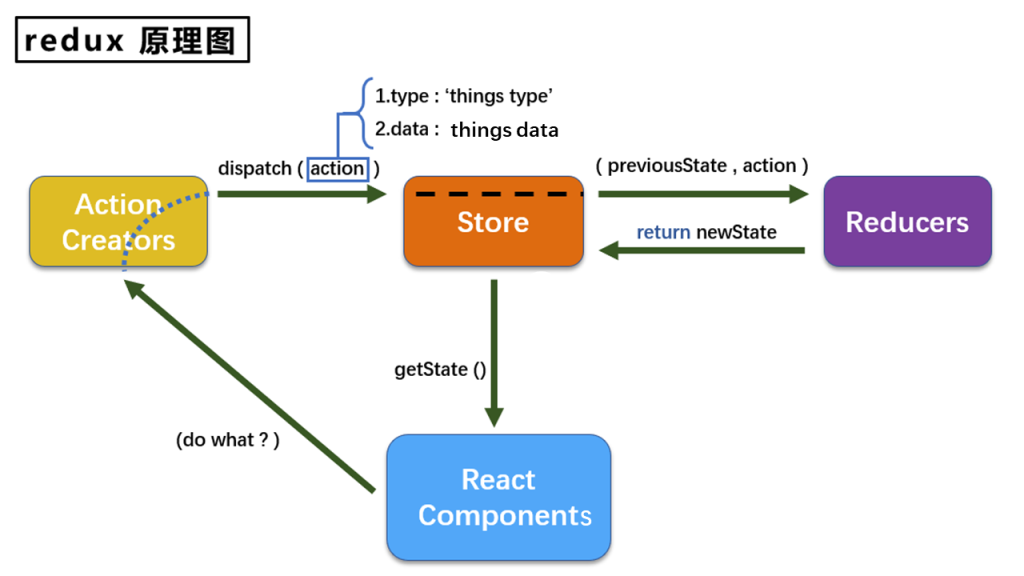
redux 的三个核心概念
action
- 动作的对象
- 包含2个属性
- type:标识属性, 值为字符串, 唯一, 必要属性
- data:数据属性, 值类型任意, 可选属性
- 例子:
{ type: 'ADD_STUDENT',data:{name: 'tom',age:18} }
reducer
- 用于初始化状态、加工状态。
- 加工时,根据旧的
state
和action
, 产生新的state
的纯函数。
store
- 将
state
、action
、reducer
联系在一起的对象 - 如何得到此对象?
import {createStore} from 'redux'
import reducer from './reducers'
const store = createStore(reducer)
- 此对象的功能?
getState()
: 得到state
dispatch(action)
: 分发action
, 触发reducer
调用, 产生新的state
subscribe(listener)
: 注册监听, 当产生了新的state
时, 自动调用
redux 的核心 API
createstore()
作用:创建包含指定 reducer
的 store
对象
store
对象
- 作用:
redux
库最核心的管理对象 - 它内部维护着:
state
reducer
- 核心方法:
getState()
dispatch(action)
subscribe(listener)
- 具体编码:
store.getState()
store.dispatch({type:'INCREMENT', number})
store.subscribe(render)
applyMiddleware()
作用:应用上基于 redux 的中间件(插件库)
combineReducers()
作用:合并多个 reducer
函数
redux 只负责管理状态,至于状态的改变驱动着页面的展示,要靠我们自己写
// 安装 redux npm i redux // store.js import { legacy_createStore as createStore } from 'redux' import countReducer from './count_reducer' export default createStore(countReducer) // count_reducer.js const initState = 0 export default function countReducer (prevState = initState, action) { const { type, data } = action switch (type) { case 'increment': return prevState + data case 'decrement': return prevState - data default: return prevState } } // 使用 import store from '../../redux/store' // 检测 redux 状态的变化 store.subscribe(() => { console.log('redux 变化了') this.setState({}) }) } // or // index.js store.subscribe(() => { ReactDOM.render(<App />, document.getElementById('root')) }) store.dispatch({ type: 'increment', data: 1 })
redux
store.js
constant.js
count_reducer.js
count_action.js
redux 异步 action
- 明确:延迟的动作不想交给组件自身,想交给 action
- 何时需要异步 action:想要对状态进行操作,但是具体的数据靠异步任务返回
- 具体编码:
- 安装
npm i redux-thunk
,并配置在 store 中
import { legacy_createStore as createStore, applyMiddleware } from 'redux'
import countReducer from './count_reducer'
import thunk from 'redux-thunk'
export default createStore(countReducer, applyMiddleware(thunk))
- 创建 action 的函数不再返回一般对象,而是一个函数,该函数中写异步任务
import { INCREMENT, DECREMENT } from './constant'
export const createIncrementAction = (data) => ({ type: INCREMENT, data })
export const createDecrementAction = (data) => ({ type: DECREMENT, data })
export const createDecrementAsyncAction = (data, time) => {
return (dispatch) => {
setTimeout(() => {
dispatch(createIncrementAction(data))
}, time)
}
}
- 异步任务有结果后,分发一个同步的 action 去真正操作数据
异步 action 不是必须要写的,完全可以自己等待异步任务的结果了再去分发同步 action
正文完